LINE Messaging APIを活用―テキスト以外のメッセージを送信する方法
作成日:2023.06.07
PHPを使って画像や位置情報、テンプレートなど、テキスト以外のメッセージをLINEユーザーに送信する手法を紹介します。
error この記事は最終更新日から1年以上が経過しています。
PHPでLINEにテキスト以外のメッセージオブジェクトを送ってみる。
参考: メッセージオブジェクト (Messaging APIリファレンス)
画像メッセージ
$messages = array();
$messages[0] = array(
'type' => 'image',
// オリジナルの画像URL
'originalContentUrl' => 'https://www.abe-tatsuya.com/image/original.png',
// プレビュー用画像URL
'previewImageUrl' => 'https://www.abe-tatsuya.com/image/preview.png',
);
$data = array(
'to' => 'my_user_id', //送信先のLINEユーザーID
'messages' => $messages,
);
$json_data = json_encode($data);
$url = 'https://api.line.me/v2/bot/message/push';
$api_key = 'LINE_ACCESS_TOKEN'; // LINE API のACCESS TOKEN
$headers = array(
'Content-Type: application/json',
"Authorization: Bearer {$api_key}"
);
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $json_data);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
$apiresult = json_decode($response);
var_dump($apiresult);
これで単純に画像を送れる。
位置情報メッセージ
$messages = array();
$messages[0] = array(
'type' => 'location',
'title' => '橿原神宮前駅',
'address' => '〒634-0063 奈良県橿原市久米町618',
'latitude' => '34.4834076',
'longitude' => '135.7938065',
);
$data = array(
'to' => 'my_user_id', //送信先のLINEユーザーID
'messages' => $messages,
);
$json_data = json_encode($data);
$url = 'https://api.line.me/v2/bot/message/push';
$api_key = 'LINE_ACCESS_TOKEN'; // LINE API のACCESS TOKEN
$headers = array(
'Content-Type: application/json',
"Authorization: Bearer {$api_key}"
);
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $json_data);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
$apiresult = json_decode($response);
var_dump($apiresult);
位置情報とGoogle Mapsへのリンクを送る。
ボタンテンプレート
$messages = array();
$messages[0] = array(
'type' => 'template',
'altText' => 'メニューリスト',
'template' => array(
'type' => 'buttons',
'thumbnailImageUrl' => 'https://www.abe-tatsuya.com/img/thumbnail.png',
'imageAspectRatio' => 'square',
'title' => 'メニューリスト',
'text' => 'ご注文を以下の2つから選んでください。',
'actions' => array(
0 => array(
'type' => 'postback',
'label' => 'フライドポテト',
'data' => 'select_0',
'displayText' => 'フライドポテトを注文する。',
),
1 => array(
'type' => 'postback',
'label' => 'フライドオニオン',
'data' => 'select_1',
'displayText' => 'フライドオニオンを注文する。',
),
2 => array(
'type' => 'uri',
'label' => 'ググる',
'uri' => 'https://google.com/',
'displayText' => 'Googleで検索する。',
),
),
),
);
$data = array(
'to' => 'my_user_id', //送信先のLINEユーザーID
'messages' => $messages,
);
$json_data = json_encode($data);
$url = 'https://api.line.me/v2/bot/message/push';
$api_key = 'LINE_ACCESS_TOKEN'; // LINE API のACCESS TOKEN
$headers = array(
'Content-Type: application/json',
"Authorization: Bearer {$api_key}"
);
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $json_data);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
$apiresult = json_decode($response);
var_dump($apiresult);
複数のボタンつきのテンプレート。サムネもつけれる。ボタンは最大4つ。
確認テンプレート
$messages = array();
$messages[0] = array(
'type' => 'template',
'altText' => '注文をキャンセルしますか?',
'template' => array(
'type' => 'confirm',
'text' => '注文をキャンセルしてもよろしいですか?',
'actions' => array(
0 => array(
'type' => 'postback',
'label' => 'はい',
'data' => 'select_0',
'displayText' => 'はい',
),
1 => array(
'type' => 'postback',
'label' => 'いいえ',
'data' => 'select_1',
'displayText' => 'いいえ',
),
),
),
);
$data = array(
'to' => 'my_user_id', //送信先のLINEユーザーID
'messages' => $messages,
);
$json_data = json_encode($data);
$url = 'https://api.line.me/v2/bot/message/push';
$api_key = 'LINE_ACCESS_TOKEN'; // LINE API のACCESS TOKEN
$headers = array(
'Content-Type: application/json',
"Authorization: Bearer {$api_key}"
);
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $json_data);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
$apiresult = json_decode($response);
var_dump($apiresult);
二択の確認ボタンを表示するテンプレート。
カルーセルテンプレート
$messages = array();
$messages[0] = array(
'type' => 'template',
'altText' => 'どのメニューを注文しますか?',
'template' => array(
'type' => 'carousel',
'columns' => array(
0 => array(
'thumbnailImageUrl' => 'https://www.abe-tatsuya.com/img/thumbnail_0.png',
'imageAspectRatio' => 'square',
'title' => 'フライドポテト',
'text' => 'じゃがいもを揚げた美味しいやつです。',
'actions' => array(
0 => array(
'type' => 'postback',
'label' => 'フライドポテトを注文する',
'data' => 'select_0',
'displayText' => 'フライドポテトを注文する',
),
1 => array(
'type' => 'uri',
'label' => 'フライドポテトについて調べる',
'uri' => 'https://google.com/',
'displayText' => 'フライドポテトについて調べる',
),
),
),
1 => array(
'thumbnailImageUrl' => 'https://www.abe-tatsuya.com/img/thumbnail_1.png',
'imageAspectRatio' => 'square',
'title' => 'フライドオニオン',
'text' => 'たまねぎを揚げた美味しいやつです。',
'actions' => array(
0 => array(
'type' => 'postback',
'label' => 'フライドオニオンを注文する',
'data' => 'select_1',
'displayText' => 'フライドオニオンを注文する',
),
1 => array(
'type' => 'uri',
'label' => 'フライドオニオンについて調べる',
'uri' => 'https://google.com/',
'displayText' => 'フライドオニオンについて調べる',
),
),
),
),
),
);
$data = array(
'to' => 'my_user_id', //送信先のLINEユーザーID
'messages' => $messages,
);
$json_data = json_encode($data);
$url = 'https://api.line.me/v2/bot/message/push';
$api_key = 'LINE_ACCESS_TOKEN'; // LINE API のACCESS TOKEN
$headers = array(
'Content-Type: application/json',
"Authorization: Bearer {$api_key}"
);
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $json_data);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
$apiresult = json_decode($response);
var_dump($apiresult);
ボタンテンプレートをカルーセル表示できる処理。
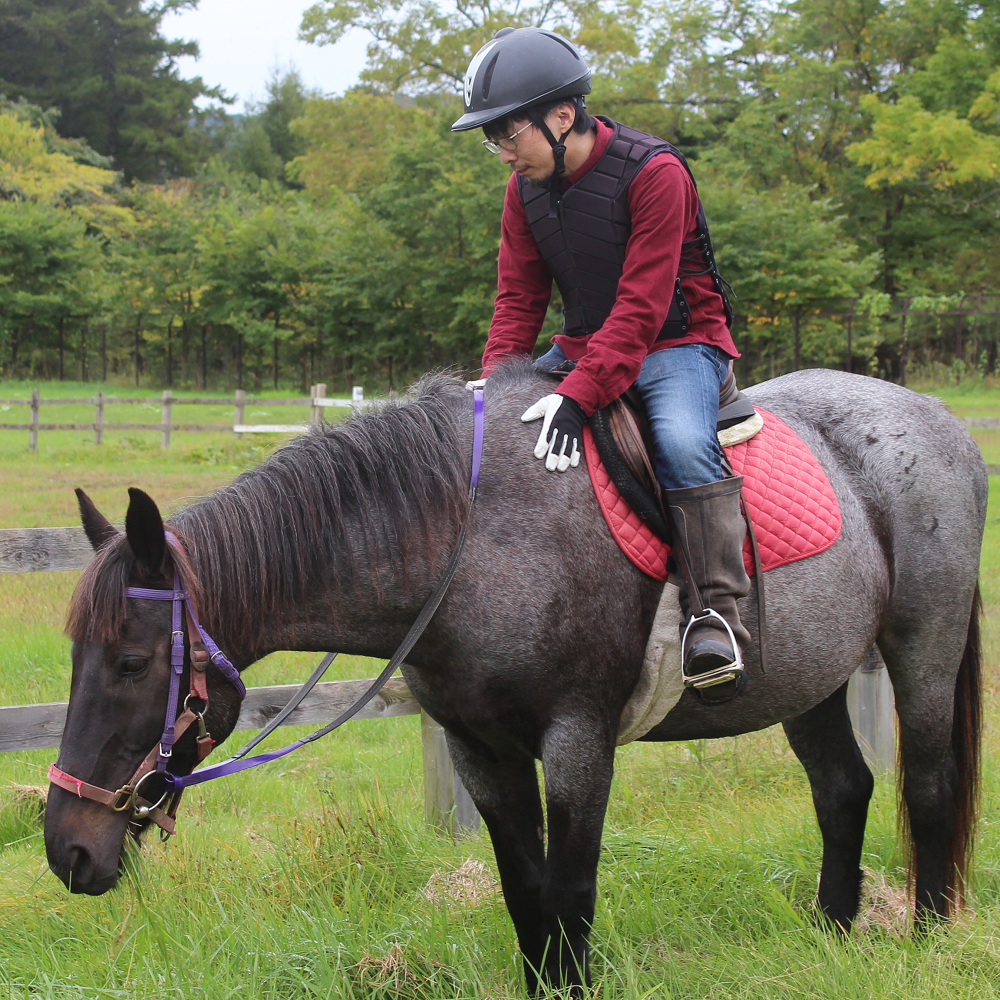
奈良市を拠点に、25年以上の経験を持つフリーランスWebエンジニア、阿部辰也です。
これまで、ECサイトのバックエンド開発や業務効率化システム、公共施設の予約システムなど、多彩なプロジェクトを手がけ、企業様や制作会社様のパートナーとして信頼を築いてまいりました。
【制作会社・企業様向けサポート】
Webシステムの開発やサイト改善でお困りの際は、どうぞお気軽にご相談ください。小さな疑問から大規模プロジェクトまで、最適なご提案を心を込めてさせていただきます。
ぜひ、プロフィールやWeb制作会社様向け業務案内、一般企業様向け業務案内もご覧くださいね。
LINEメッセージをWebhook経由で取得するシンプルなPHPコード
2023.11.20
LINE Messaging APIを使って、Webhookで送信されたメッセージを取得するPHPスクリプトの基本テンプレートをご紹介します。
LINE Messaging APIでPHPを使ったメッセージ送信入門
2023.06.06
LINE Messaging APIを使い、PHPでテキストメッセージを送信する手順を解説します。
CodeIgniter 4 フォーム入力バリデーション入門 ― 基本操作とカスタマイズ手法
2025.04.06
CodeIgniter 4でのフォーム入力値取得とバリデーション処理を、シンプルな実装例を通して解説します。基本的なルール設定から、エラーメッセージの出力、フォームラベルの日本語化やローカライズ方法まで、実践的なテクニックをコンパクトにまとめています。
PHPによる OpenAI TTS API 実装ガイド ― GPT-4o mini TTSでテキストを音声に変換
2025.04.05
本記事では、最新の OpenAI TTS モデル「GPT-4o mini TTS」を利用し、PHPでテキストを自然な音声に変換する実装手法を詳しく解説します。curl を用いたリクエスト送信、JSON形式のパラメータ設定、ファイル出力処理まで、実際のサンプルコードを交えながら、各工程のポイントとパラメータの意味について解説。